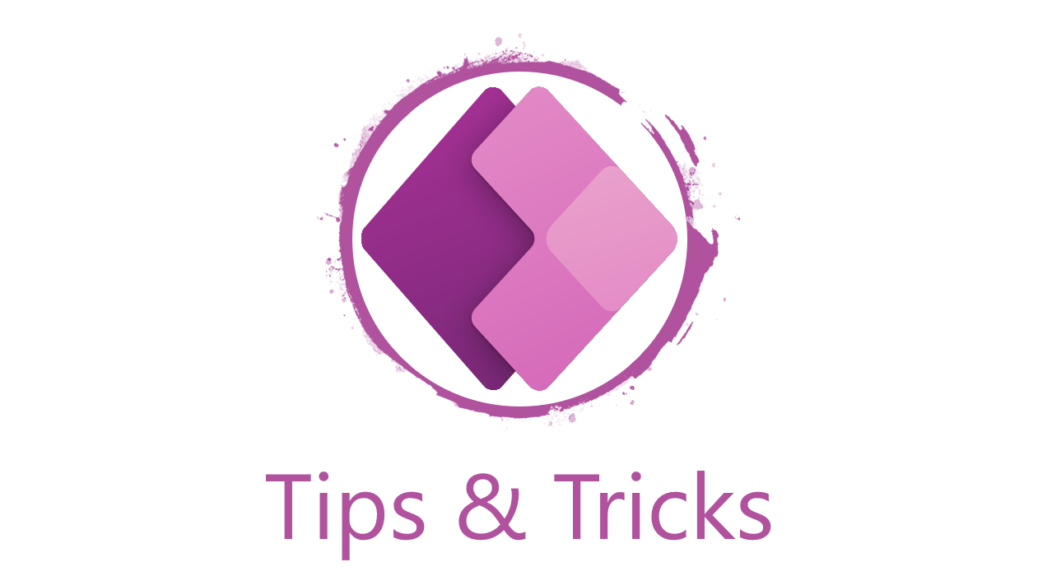
Power Apps – Error Handling for APIs
Custom Connectors and APIs are a pleasure for Power Apps. I can access my needed data, the setup is very fast, and finally I can use the methods of my connector from my Power App. This works fine, except when my API call fails.
How can I handle such errors in my PowerApps? Is there something like a Try-Catch-Block?
To be honest, it took me a while to find a setup that works for me. So let me first explain my API to describe my problem…
Custom Connector API Definition
My API provide a “get” method with a Path-Parameter “list” (name of my list). Furthermore, my API returns the count and the list of records as “value” in the response (HTTP 200). Therefore, my Swagger API Definition in my custom connector is:
swagger: '2.0'
paths:
/data/{list}:
get:
summary: GetData
operationId: GetData
parameters:
- {name: list, in: path, type: string, required: true}
responses:
'200':
description: 'Success'
schema:
type: object
properties:
count: {type: integer, format: int32, description: count}
value:
type: array
items:
type: object
properties:
id: {type: string, description: id}
value: {type: string, description: value}
This works fine for common lists. In case of my “special-list” as parameter “list” it will fail and raise an error. As result my response Http-Status Code of my API call is HTTP 400.
GET http://localhost:7071/api/data/special-list
HTTP/1.1 400 Bad Request
Connection: close
Date: Sun, 28 Feb 2021 11:35:07 GMT
Content-Type: text/plain; charset=utf-8
Server: Kestrel
Transfer-Encoding: chunked
Bad Request
This means I have to add another response to my API-Definition. The result is:
swagger: '2.0'
paths:
/data/{list}:
get:
summary: GetData
operationId: GetData
parameters:
- {name: list, in: path, type: string, required: true}
responses:
'200':
description: 'Success'
# schema: ...
'400':
description: 'Bad Request'
Using the API
Now it’s time to describe my demo Power App. The Power App contains a Drop Down control to select a list name. Secondly I have added some Label controls to display count, success status, and provides elements from “value” in a Data Table. Finally I have added a Button control to invoke my API.
My button “Get Data” store the result of my API in a local variable as follows:
OnSelect: |-
=UpdateContext({result: 'Demo-API'.GetData(edListNames.Selected.Value)});
Everything works fine until I call my API with the parameter “special-list”. This is because my API does not provide a valid result and responde with HTTP Status 400 “Bad Request”.
The Power Apps Monitor shows the expected behavior in the current session:
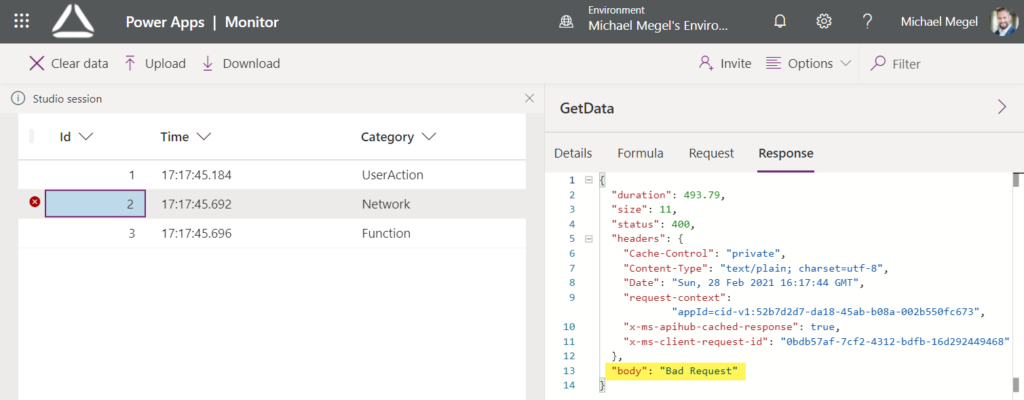
So far so good, for this reason I have already added the second response to my API. Let’s switch back to the Power Apps Designer and see the result…
Power Apps Designer and App Checker
I thought, this shouldn’t be a problem for my Power App. But unfortunately it is, because my Power Apps Designer regenerate the data structure of my used variable and shows now an error:
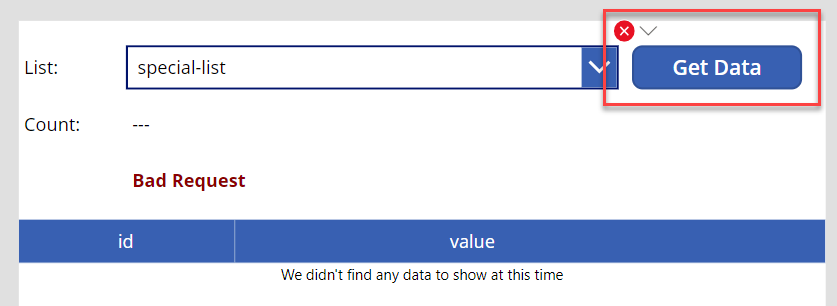
When I inspect the formula I see the correct response (“Bad Request”). Moreover, I can not handle the response – I have no access to the Status Code.
Furthermore the App Checker detect also the problem and shows also the found error:
Such a calculation error is not a problem in my tiny Power App example. But I know from my experience that this becomes a nightmare in larger Power Apps with many controls and complex UI customizations.
The result is, my autocompletion and the formula resolution will fail and generate other issues. This can lead to a total failure of the entire Power App and makes further development impossible.
In conclusion: I have to fix this Error!
Error Handling
Again, my goal is to call my API, receive data and store the result in a variable. Moreover I want to avoid breaking formulas, when my API call fails with any error or unexpected response.
Microsoft provides two important functions to handle Errors in Power Apps. These are IsError(…) and IfError(…). Furthermore this “experimental features” must be activated in my PowerApp because:
“… IfError and IsError functions are part of an experimental feature…” and “… Formula-level error management experimental feature in advanced settings must be turned on…” (Microsoft Documentation)
Now I can use the “IfError” function like a Try-Catch-Block. My changed code of button “Get Data” is now:
OnSelect: |-
=IfError(
// TRY
UpdateContext({result: Patch('Demo-API'.GetData(edListNames.Selected.Value), {success: true})});
,
// CATCH
UpdateContext({result: {count: Blank(), value: Blank(), success: false}});
);
As you can see, I have added the element “success” to detect a successful or failing API response. In case of a successful call, I need to “Patch(…)” my result and add the “success: true” information.
Now my Power App accept also the “Bad Result” response from my API without any issues:
In addition, my Power Apps Designer show no longer an error. This is because the data structure of my local variable isn’t changed anymore.
Summary
My short example have shown, how you can use the experimental feature “IfError(…)” to handle unexpected results and errors from your API. In other words, you can use it like a Try-Catch-Block. With that you are able to react on issues during an API call. Moreover, this helps a lot to protect the data structure and integrity of your Power App.
But there is also a downside. My setup do not detect the reason of the failing call. In detail, you have no access to the Http-Response Status Code. Because of this, you will not be able to distinguish between an HTTP 400, HTTP 404, HTTP 500 and other issues.
Personally, I see more advantages in catching the whole error. I need a Power Apps Designer without errors. This allows a correct formula resolution. As result, my autocompletion of variables works during development much more stable. Finally, I can also analyze API errors in the Power Apps Monitor or by using Application Insights …but that’s another story…